Go expert
from basics to gRPC
and heavy-duty APIs
and heavy-duty APIs
Master Go (Golang) at a professional level - from syntax to creating powerful APIs with gRPC and
Protocol Buffers!
✔ No “water” - only practice, real cases and projects.
✔ For beginners and experienced - you can start from scratch or deepen your knowledge.
✔ Up-to-date 2025 program - learn what you really need in the industry.
Start now
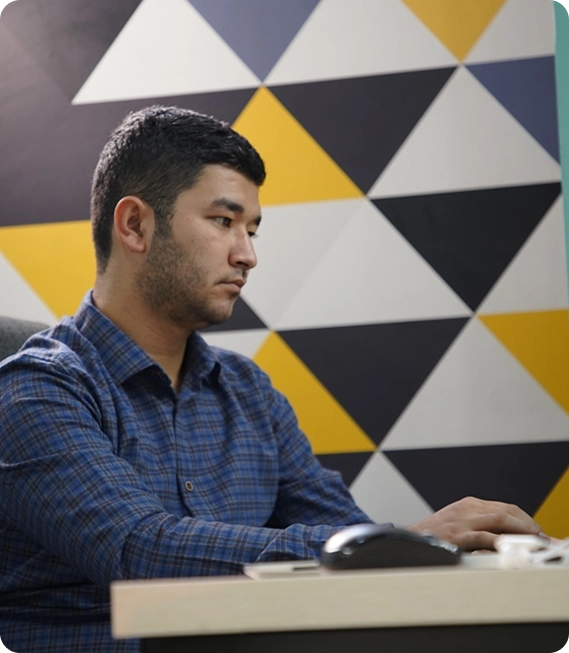